What is the merge sort algorithm?
Merge sort is one of the most efficient sorting algorithms. It is based on the divide-and-conquer strategy. Merge sort continuously cuts down a list into multiple sublists until each has only one item, then merges those sublists into a sorted list. How Does the Merge Sort Algorithm Work? Merge sort algorithm can be executed in two ways:
What is the best case complexity of merge sort?
Best Case Complexity – It occurs when there is no sorting required, i.e. the array is already sorted. The best-case time complexity of merge sort is O (n*logn). Average Case Complexity – It occurs when the array elements are in jumbled order that is not properly ascending and not properly descending.
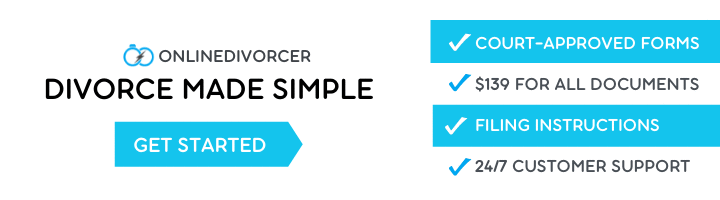
How do you sort an array by comparing and merging?
Now merge the two arrays of single elements, by comparing all the numbers in one block with all the elements in the another block and the elements are placed accordingly in the merged block based on ascending order sorting obtained by comparison. Now this method of sorting elements by comparing and merging has to be done recursively.
What is the difference between merge sort and conquer sort?
Merge sort 1 If A Contains 0 or 1 elements then it is already sorted, otherwise, Divide A into two sub-array of equal number of… 2 Conquer means sort the two sub-arrays recursively using the merge sort. 3 Combine the sub-arrays to form a single final sorted array maintaining the ordering of the array. More

Merge sort is one of the most efficient sorting algorithms. It works on the principle of Divide and Conquer. Merge sort repeatedly breaks down a list into several sublists until each sublist consists of a single element and merging those sublists in a manner that results into a sorted list.
What is merge sort explain with example?
Merge sort. An example of merge sort. First, divide the list into the smallest unit (1 element), then compare each element with the adjacent list to sort and merge the two adjacent lists. Finally, all the elements are sorted and merged.
How does merge sort work step by step?
Here’s how merge sort uses divide-and-conquer:
- Divide by finding the number q of the position midway between p and r.
- Conquer by recursively sorting the subarrays in each of the two subproblems created by the divide step.
- Combine by merging the two sorted subarrays back into the single sorted subarray array[p..
What is merge sort program in C?
Advertisements. Merge sort is a sorting technique based on divide and conquer technique. With the worst-case time complexity being Ο(n log n), it is one of the most respected algorithms.
Why we use merge sort?
Merge Sort is useful for sorting linked lists. Merge Sort is a stable sort which means that the same element in an array maintain their original positions with respect to each other. Overall time complexity of Merge sort is O(nLogn). It is more efficient as it is in worst case also the runtime is O(nlogn)
What is merge sort application?
Applications. Merge Sort is useful for sorting linked lists in O(n Log n) time. Merge sort can be implemented without extra space for linked lists. Merge sort is used for counting inversions in a list. Merge sort is used in external sorting.
How many times is merge sort called?
Since each recursive step is one half the length of n . Since we know that merge sort is O(n log n) could stop here as MergeSort is called log n times, the merge must be called n times.
Why merge sort algorithm is used?
What are the advantages of merge sort?
What Are the Advantages of the Merge Sort?
- Merge sort can efficiently sort a list in O(n*log(n)) time.
- Merge sort can be used with linked lists without taking up any more space.
- A merge sort algorithm is used to count the number of inversions in the list.
- Merge sort is employed in external sorting.